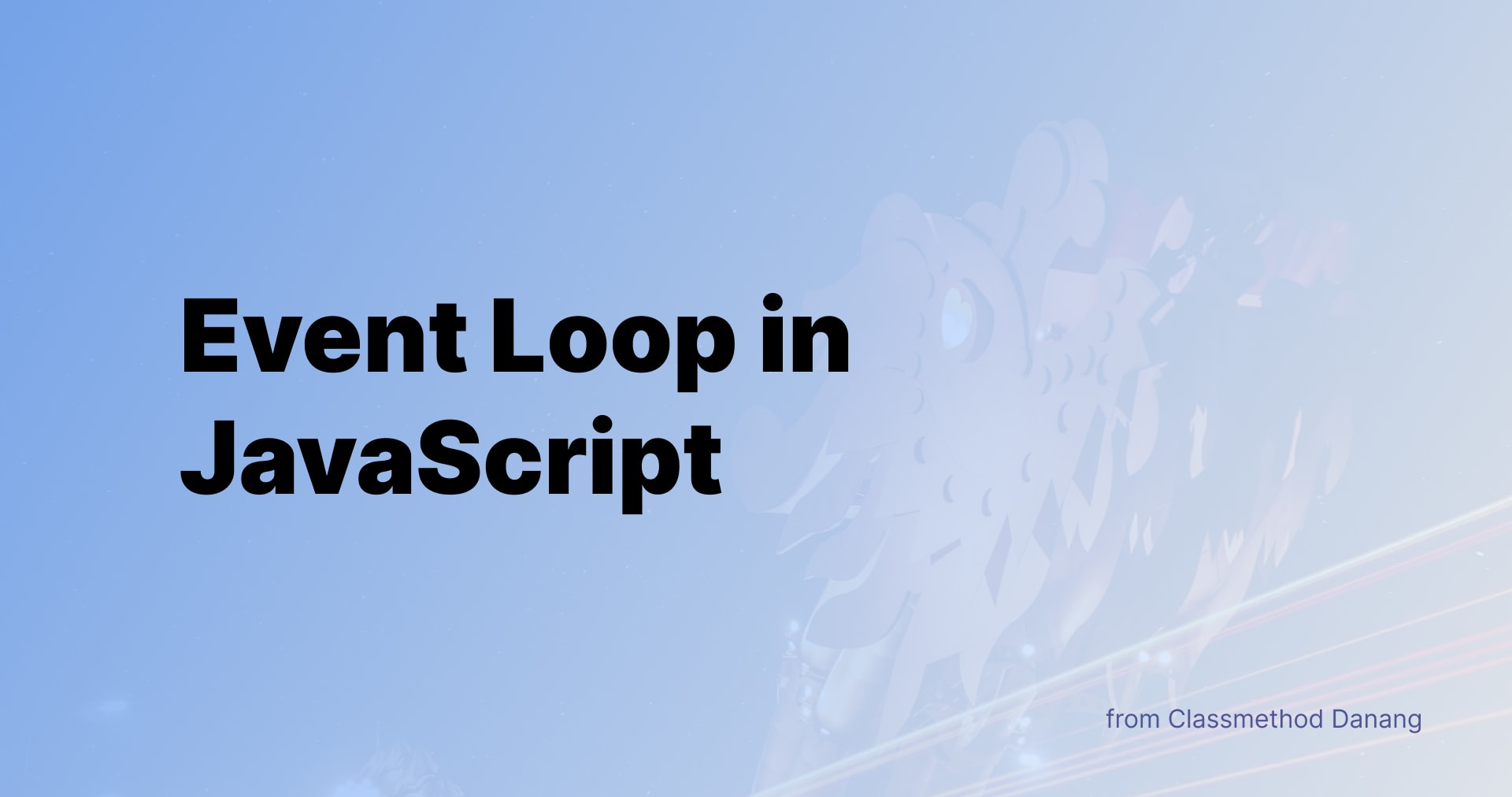
Understanding the Event Loop in JavaScript
The Event Loop is a fundamental concept in JavaScript that enables efficient execution of asynchronous tasks in a single-threaded environment. By managing tasks through the Call Stack, Web APIs, and Callback Queue, JavaScript ensures non-blocking execution, optimizing performance and responsiveness. This article explores the Event Loop's workings, its components, real-world examples, and its impact on JavaScript performance.
Introduction
JavaScript is a single-threaded programming language, which means it can execute one task at a time on the main thread. This limitation raises challenges when handling long-running or asynchronous tasks like network requests or file reading. Without mechanisms like the Event Loop, such tasks would block subsequent operations, leading to poor performance and frustrating user experiences.
The Event Loop provides a way to execute tasks asynchronously while keeping the application responsive. It is at the heart of JavaScript's runtime and ensures smooth coordination between synchronous and asynchronous code execution.
Synchronous and Asynchronous Execution
Synchronous Execution
In synchronous programming, tasks are executed one after the other, blocking subsequent tasks until the current one is completed. This behavior is straightforward but can cause issues when handling long tasks.
Example:
console.log("Task 1");
console.log("Task 2");
console.log("Task 3");
Output:
Task 1
Task 2
Task 3
In this example, each console.log
statement waits for the previous one to finish before executing.
Asynchronous Execution
Asynchronous programming allows JavaScript to handle tasks without blocking the main thread. Tasks such as API calls or timers are delegated to Web APIs, processed in the background, and queued for execution once the Call Stack is clear.
Example:
console.log("Start");
setTimeout(() => console.log("Async Task"), 2000);
console.log("End");
Output:
Start
End
Async Task
Here, the setTimeout
function delegates the task to the Web API, allowing console.log("End")
to execute without waiting. The callback is executed once the timer completes.
What is the Event Loop?
The Event Loop is the mechanism that ensures non-blocking, asynchronous operations in JavaScript. It coordinates the execution of synchronous and asynchronous tasks by managing the Call Stack, Web APIs, and Callback Queue.
Core Components of the Event Loop
1. Call Stack
The Call Stack is where JavaScript keeps track of function calls. Each new function call is added to the stack and removed once executed.
Example:
function bar() {
return "bar";
}
function foo() {
return bar() + " foo";
}
console.log(foo());
Execution Flow:
foo()
is added to the Call Stack.- Inside
foo()
,bar()
is added, executed, and removed. - Finally,
foo()
completes and is removed.
2. Web APIs
Web APIs handle asynchronous tasks like timers (setTimeout), AJAX requests, and DOM events. These tasks are processed outside the Call Stack and are queued for execution once completed.
Example:
console.log("Start");
setTimeout(() => console.log("Timer task"), 2000);
console.log("End");
Output:
Start
End
Timer task
3. Callback Queue
The Callback Queue stores functions ready to be executed once the Call Stack is empty. Tasks like resolved promises and setTimeout callbacks wait here before execution.
Illustration of the Event Loop
Below are visual representations of the Event Loop:
- The Call Stack processes the setTimeout function, delegating it to Web APIs.
- The Web APIs manage tasks like timers and send the callback to the Callback Queue when ready.
- The Event Loop ensures the callback is moved to the Call Stack for execution once it is clear.
How It Works?
- The Event Loop monitors the Call Stack and the Callback Queue.
- When the Call Stack is empty, it moves the next task from the Callback Queue to the Call Stack for execution.
- Tasks originating from Web APIs (e.g., setTimeout, AJAX) are processed asynchronously and queued in the Callback Queue when ready.
- This process ensures non-blocking execution and keeps JavaScript responsive.
Example 1: Timer Function
console.log("Task 1");
setTimeout(() => console.log("Task 2"), 1000);
console.log("Task 3");
Output:
Task 1
Task 3
Task 2
Execution Flow:
- Task 1 and Task 3 are executed immediately in the Call Stack.
setTimeout
delegates Task 2 to the Web API.- After 1000 ms, Task 2 moves to the Callback Queue and waits for the Call Stack to clear.
Example 2: Fetch API
console.log("Fetching data...");
fetch("https://jsonplaceholder.typicode.com/users")
.then(response => console.log("Data received"))
.catch(error => console.error("Error occurred"));
console.log("Fetch initiated");
Output:
Fetching data...
Fetch initiated
Data received
Execution Flow:
- Logs Fetching data... and Fetch initiated in the Call Stack.
- Fetch operation is sent to Web APIs.
- Once resolved or rejected, .then or .catch moves to the Callback Queue.
Conclusion
The Event Loop is essential for writing performant JavaScript code. By mastering the Call Stack, Web APIs, and Callback Queue, developers can ensure their applications are responsive, efficient, and free from blocking issues. Applying this knowledge to real-world scenarios, such as handling timers and asynchronous operations, allows for building robust and scalable applications.
References